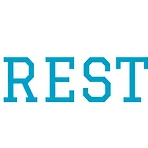
해당 글은 02-RESTful-API-예제-Spring-Framework 에서 작성된 프로젝트를 좀 더 REST 하게 변경하는 과정을 간략하게 담은 글입니다. 이러한 과정을 통해 리처드슨 성숙도 모델의 3 level에 가까운 혹은 만족하는 API를 구현할 수 있습니다. ETag란 무엇인가요? (Entity Tag) HTTP Spec의 일부로써 웹 캐시 유효성 검사를 위해 제공하는 메커니즘 중 하나입니다. 이 방식의 특징으로는 클라이언트가 조건에 따른 요청을 할 수 있게끔 한다는 것입니다. 리소스에 대한 특정 버전 혹은 결과에 대해 ETag를 생성하여 요청을 검증하고, 서버 리소스에서 변경이 발생한다면 ETag를 변경하여 검증을 실패하게 합니다. 이는 새로운 리소스 결과를 클라이언트에 전달하며 ETag 값..
Programming
2021. 3. 7. 23:00
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- URI
- Global Cache
- THP
- URN
- 근황
- rabbitmq
- java
- RESTful
- JDK Dynamic Proxy
- configuration
- 게으른 개발자 컨퍼런스
- Cache Design
- 게으른개발자컨퍼런스
- Switch
- Data Locality
- RPC
- cglib
- HTTP
- JVM
- mybatis
- AMQP
- hypermedia
- spring
- Local Cache
- Distributed Cache
- 소비자 관점의 api 설계 패턴과 사례 훑어보기
- JPA
- Url
- lambda
- spring AOP
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
글 보관함