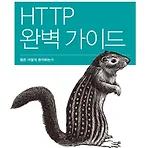
전 세계의 브라우저, 서버, 웹 애플리케이션은 모두 HTTP를 통해 서로 대화한다 HTTP : 인터넷의 멀티미디어 배달부 HTTP 는 신뢰성 있는 데이터 전송 프로토콜을 사용하기 때문에, 데이터가 지구 반대편에서 오더라도 전송 중 손상되거나 꼬이지 않음을 보장한다. 이 덕분에 사용자는 인터넷에서 얻는 정보가 손상된 게 아닌지 염려하지 않아도 된다. 웹 클라이언트와 서버 웹 컨텐츠는 서버에 존재한다. 서버는 보통 HTTP 프로토콜로 의사소통하기 때문에 HTTP 서버라고 부르기도 한다. 웹 서버는 인터넷의 데이터를 저장하고 클라이언트가 요청한 데이터를 제공한다. 웹 클라이언트 크롬 익스플로러 사파리 등 리소스 웹 서버는 "리소스"라는 것을 관리하고 제공한다. 리소스란? 웹 서버의 정적 파일 (HTML, Te..
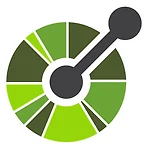
Open API Specfication 3? OAS는 RESTful API에 대한 정보들을 정의하여 소스 코드에 접근하지 않아도 서비스의 기능을 검색, 확인하고 사용할 수 있게끔 지원해주는 표준 인터페이스이다. OAS 3는 2017년 7월에 발표된 spec이며, 많은 개선이 이루어졌다고 한다. 해당 spec은 JSON, YAML을 통하여 작성할 수 있다. documentation generation tools를 사용하여서 해당 API의 기능, 테스트 코드 등을 사용자(클라이언트 개발자) 입장에서 쉽게 알 수 있게 해 준다. 스프링에서 사용할 수 있는 OAS 라이브러리 Spring fox : OAS 2를 지원하는 라이브러리이다. Springdoc-openapi v1.5.1 : OAS 3을 지원하는 라이브러..
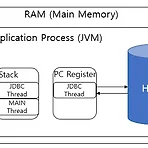
H2의 Local, Server 개념 Embedded 모드 H2 DB를 시스템의 메인 메모리에서 (JVM 위에서) 구동시키는 방식으로 application이 종료된다면 저장, 수정된 Data가 손실(휘발) 된다. 즉 기본적으로는 영속적이지 않은 방식이다. → 데이터에 대한 영속성을 제공하는 방법은 존재한다. 메인 메모리에 DB를 띄워놓고 해당 DB를 사용하는 Application의 스레드로 데이터에 바로 접근함으로써 데이터 읽기, 쓰기에 대한 성능을 향상할 수 있으므로 유용하게 사용할 수 있으며, 데이터 캐싱 DB에 대해서도 H2를 고려할 수 있다고 한다. 하지만 JVM에서 데이터 연산에 사용되는 쓰레드를 인터럽트 하지 않을 수 있기에, IO 수행 시에 I/O Handler가 닫힘으로써 데이터베이스의 손..
Post Entity, DTO 들을 만들고 생성 테스트를 진행한 뒤에 Post Controller와 Service를 생성하고 PostMapper에 Mapper 어노테이션 작성과 Mapper.xml 작성을 완료한 후 Teliend API를 통한 테스트를 진행하였다. 그런데.. POST 요청 보냈더니 이러한 에러가 발생하였다. org.apache.ibatis.binding.BindingException: Invalid bound statement (not found): com.somaeja.post.mapper.PostMapper.save at org.apache.ibatis.binding.MapperMethod$SqlCommand.(MapperMethod.java:235) ~[mybatis-3.5.5.ja..
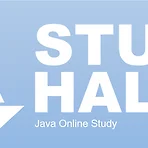
4주 차 시작합니다! 선택문 (switch)? 주어진 조건 값의 결과에 따라 프로그램이 다른 명령을 수행하도록 하는 일종의 조건문이다. 모든 값, 범위를 기반으로 판단하는 if 문과 달리 정수 값이나 열거된 값 또는 문자, 문자열만을 사용할 수 있다. 컴파일러를 통해 실행 경로를 설정하는 점프 테이블이라는 것이 만들어지게 되어서 많은 조건을 비교하여야 할 때, if else 보다 더 빠른 성능을 보이게 된다. → case의 수가 5개 이상이라면, 성능 차이가 보이기 시작한다. if else에 비하여서 좋은 가독성을 가지고 있다. switch 문 public static String monthCheck(int num){ int days = 0; switch (num) { case 1 : case 3 : c..
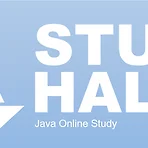
3주 차 시작합니다! 연산이란? 주어진 정보를 통해 일정한 규칙에 따라 어떤 값이나 결과를 구하는 과정을 의미한다. 연산자란? 연산을 진행하는 동안 사용되는 기호를 말한다. 연산 대상의 수나 연산 방식에 따라서 명칭이 나뉘게 된다. 피연산자란? 연산될 대상을 말하며. 변수, 상수, 리터럴 등을 의미한다. 단항 연산자 (Unary Operator) 연산이 수행될 피연산자가 1개인 경우 해당 연산자를 단항 연산자라고 한다. 전위 증감, 후위 증감 연산자 단항으로써 사용하는 +, - 연산자 (부호 연산자) 비트 반전 ~ 연산자 이항 연산자 (Binary Operator) 연산이 수행될 피연산자가 2개인 경우 해당 연산자를 이항 연산자라고 한다. 산술 연산자 비트 연산자 관계 연산자 대입 연산자 논리 연산자 삼..
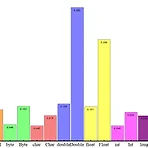
Primitive Type 종류와 값의 범위 그리고 기본 값 Primitive Variable 이란? 어떠한 Instance의 참조 값을 가지지 않고, 지정된 Type에 맞는 리터럴을 저장한 변수를 말한다. 객체를 다루지 않기 때문에 Null을 저장할 수 없으며, 같은 타입의 변수들이 동일한 값을 다룰 때에는 Operand Stack이나 Constant Pool에 리터럴을 저장해두었다가 꺼내어 사용한다. **// 정수 타입 // JDK 7 까지는 int와 long에 대해서 Unsigned 를 지원하지 않았지만, // JDK 8 부터는 int와 long에 대하여서 지원하기 시작하였다.** // 1 byte (8 bit) // -128 (-2^7) ~ 127 (2^7–1). // 기본 값은 0. (공통적으로..
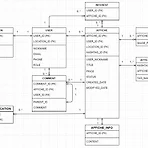
Somaeja 모든 사람이 소매상이 될 수 있는 거래 플랫폼 지역 정보 기반의 물품 거래 플랫폼 RESTful API 서버 프로젝트입니다. 당근마켓과 유사한 서비스를 제공함으로써 사용자들은 설정된 지역 정보를 가지고 다른 사람들의 물품을 확인하고 구매 의사를 밝힐 수 있습니다. Kakao Oven을 이용하여 프로토타입 설계를 진행하였으며, API 서버만 집중하여 개발을 진행합니다. 프로젝트의 대략적인 인프라 구조 프로젝트 설계 정보 프로젝트 UML 구조 변경 사항 AFFICHE USER FK 를 가짐으로 USER의 NICKNAME 제외 (닉네임 변경시 처리가 불편하다.) 11.25 테이블 네이밍 변경 AFFICHE → POST (이해하기 쉬운 익숙한 네이밍이 좋다) 11.25 게시글 삭제 유무 DELET..
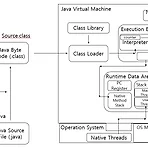
1주 차 과제 : JVM 은 무엇이며, 자바 코드는 어떻게 실행하는 것인가. JVM 이란 무엇인가 JVM (자바 가상 머신) 이란 Java와 Kotlin, Scala 등이 컴파일된 바이트코드를 실행하는 Virtual machine이다. 컴파일하는 방법 Java Compiler : wikipedia Java Compiler 명령어를 사용하고 Source File의 Path를 넘겨주면 된다. Directory\Study>Javac source.java // File Path를 이용하여 컴파일한다. -> Directory\Study\source.java 바이트코드란 무엇인가? Bytecode : wikipedia Java Bytecode 란 Java의 Execution Engine를 통해 수행될 수 있는 상태..
- Total
- Today
- Yesterday
- 게으른 개발자 컨퍼런스
- JPA
- Cache Design
- 소비자 관점의 api 설계 패턴과 사례 훑어보기
- Url
- HTTP
- AMQP
- RPC
- spring AOP
- JDK Dynamic Proxy
- Distributed Cache
- spring
- Data Locality
- java
- Local Cache
- RESTful
- configuration
- Global Cache
- JVM
- Switch
- URI
- hypermedia
- mybatis
- lambda
- 근황
- 게으른개발자컨퍼런스
- THP
- cglib
- URN
- rabbitmq
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |